Introduction
In Apache ANT Tasks following topics will be discussed:
ANT Tasks
- Compile Task
- Archive Task
- File Task
- Property Task
- Version Control Task
- JUnit Task
- Remote Task
Description
ANT has many standard tasks where the real work gets done. As Ant is extendable, many optional tasks are developed using standard tasks.They require libraries and jar files to be in the class path so that they can take advantage of libraries.
Description
Compiling the code is one of the common tasks. src directory parameter gives the java file location and directory parameter destination indicates the class file location. Directory structure in the src folder has to match the package name of classes.
[java]<javac srcdir=”$(src.dir)”
Destdir=”$(build.dir)”[/java]
There are some parameters that helps in include or exclude while compiling, they are:
[java]includes=”splessons/info/** , splessons/web/**”
excludes=”splessons/test/**”[/java]
The above parameters can be separated by comma or represented as follows:
[java]<include name=”splessons/info/**”/>
<include name=”splessons/web/**/>
<exclude name=”splessons/test/**/>[/java]
This can be done by using alternate compilers like classic, modern, jikes, jvc, kjc, gcj, sj, extJavac. To include the compiler, the parameter “compiler” has to be used.
There are also other parameters like deprecation, classpath, fork and debug to compile tasks.
[java]debug=”on”
deprecation=”on”
classpath=”junit.jar”
fork=”true”
compiler=”modern”[/java]
Depend is one of the important tasks of compile, which indicates the expiry date of classfiles and recompiles the removed class-files.
Description
The main feature of ANT Tasks is to package the files and reduce the code by using those packaged files. Java developers package the code in jar, war and ear files.
Example
Manifest file can also be included in jar files.
[java]
<target name=”jarExample1” depends=”init”>
<jar destfile=”${src.dir}/lib/app.jar” Basedir=”${classes.dir}” Excludes=”**/Test.class” Update=”true” Manifest=”${etc.dir}/MANIFEST.MF” />
</target>[/java]
Example
[java]
<?xml version="1.0" encoding="UTF-8"?>
<project name="War Example" default="war" basedir=".">
<target name="war">
<war destfile="example.war" webxml="metadata/web.xml">
<fileset dir="html"/>
<fileset dir="jsp"/>
<lib dir="libs"/>
<classes dir="classes"/>
<zipfileset dir="images" prefix="images"/>
</war>
</target>
</project>
[/java]
The above example consists of “example.war” war file, which includes web.xml file, all HTML files, all JSPs, libraries, classes and images.
Description
ANT Tasks possess library of file tasks, which helps in file manipulation and directories. In the previous lesson, mkdir is used to create a new directory like copy, concat, sync, get, move, and replace.
More Info
Copy task copies the group of files to a new location. Suppose, there are two directories:
[java]<?xml version="1.0" encoding="UTF-8"?>
<project name="Copy Example" default="copyfile" basedir=".">
<target name="copyfile">
<copy file="etc/MANIFEST.MF" tofile="backup/MANIFEST.MF"/>
</target>
</project>
[/java]
In the above example, manifest file copied from the etc directory and placed in the backup directory appeared as MANIFEST.MF.
Move task is same as the copy task but moves the READ_ME.txt file from etc to backup directory. By using fileset, this task can be applied on a group of files.
[java]<?xml version="1.0" encoding="UTF-8"?>
<project name="Move Example" default="movefile" basedir=".">
<target name="movefile">
<move file="etc/READ_ME.txt" tofile="backup/READ_ME.txt"/>
</target>
</project>
[/java]
Normally, Sync task is used to synchronize the target directory with other set of files.
[java]
<?xml version="1.0" encoding="UTF-8"?>
<project name="Sync Example" default="syncfiles" basedir=".">
<target name="syncfiles">
<sync todir="backup">
<fileset dir="etc"/>
</sync>
</target>
</project>
[/java]
Here, any changes made in the etc directory gets synced with the backup directory. Suppose create test.text file in addition to etc directory. After running the code, the backup directory also holds the test.txt file.
Get task downloads the files from any URL.
[java]
<?xml version="1.0" encoding="UTF-8"?>
<project name="Get Example" default="getfile" basedir=".">
<target name="getfile">
<get src="http://ant.apache.org/" dest="etc/index.html"/>
</target>
</project>
[/java]
index.html appears in the etc directory appears.
Replace task helps to replace the files of a string with another string in a file.
[java]<?xml version="1.0" encoding="UTF-8"?>
<project name="Replace Example" default="replacetoken" basedir=".">
<target name="replacetoken">
<replace file="etc/READ_ME.txt" token="@@@" value="This is so cool!"/>
</target>
</project>
[/java]
Replace asks the ANT to go through the READ_ME file in etc directory and replace the @@@ with text.
Concat task helps in concatenation of one or more resources into a single file. Here, the text "Hello World!" is added to the READ_ME file.
[java]
<?xml version="1.0" encoding="UTF-8"?>
<project name="Concat Example" default="concatfile" basedir=".">
<target name="concatfile">
<concat destfile="etc/READ_ME.txt" append="yes"> Hello, World!</concat>
</target>
</project>
[/java]
Not only these, there are many other file ANT Tasks.
Description
Property Elements are used to define the variables, which are reusable to strings such as directories or paths.
Examples
The example shows how to define properties within a build file.
[java]
<?xml version="1.0" ?>
<project name="JarBuild" basedir="." default="jarfile">
<target name="init" description="Initializes properties">
<property name="project.name" value="helloWorld" />
<property name="src.dir" value="src" />
<property name="build.dir" value="build" />
<property name="classes.dir" value="${build.dir}/classes" />
<property name="etc.dir" value="etc" />
</target>
<target name="prepare" description="Creates the build and classes directories" depends="init">
<mkdir dir="${classes.dir}" />
</target>
<target name="compile" description="Compiles the code" depends="prepare">
<javac srcdir="${src.dir}" destdir="${classes.dir}" />
</target>
<target name="jarfile" description="JARs the code" depends="compile">
<jar destfile="${build.dir}/${project.name}.jar" basedir="${classes.dir}" manifest="${etc.dir}/MANIFEST.MF" />
</target>
<target name="clean" description="Delete the build directory" depends="init">
<delete dir="${build.dir}" />
</target>
</project>[/java]
Built-in
Poperties
Along with the properties there are some more built-in properties like
Property |
Description |
ant.file |
Build file full location. |
ant.version |
Apache ANT Installation version. |
ant.java.version |
The version of the JDK that is used by ANT |
Basedir |
The basedir of the build file |
ant.project.name |
Project Name |
ant.project.default-target |
Current project's default target. |
ant.project.invoked-targets |
List of targets invoked in the current project. |
ant.core.lib |
Ant jar file full location. |
ant.home |
ANT Installation Home directory. |
Examples
property.xml
[java]
<?xml version="1.0" encoding="UTF-8"?>
<project name="property example" default="printproperties" basedir=".">
<property file="build.properties" />
<target name="printproperties">
<echo>Project Name: ${project.name} </echo>
<echo>Source Dir: ${src.dir} </echo>
<echo>Build Dir: ${build.dir} </echo>
<echo>Classes Dir: ${classes.dir} </echo>
<echo>Etc Dir: ${etc.dir} </echo>
</target>
</project>
[/java]
build.properties
[java]
project.name=Hello World
src.dir=src
build.dir=build
classes.dir=${build.dir}/classes
etc.dir=etc
[/java]
In the above example, the property file simply loads the build.properties file into the console and gives the output as follows:
Examples
ANT has tasks to read/write properties by interacting with the property files. A Property File can be used for setting properties even for external files.
property.xml
[java]
<?xml version="1.0" encoding="UTF-8"?>
<project name="Property File Example" default="createfile" basedir=".">
<target name="createfile">
<propertyfile file="dynamic.properties">
<entry key="date" type="date" value="now"/>
<entry key="buildnumber" type="int" default="0" operation="+"/>
</propertyfile>
</target>
</project>
[/java]
When the above xml file is executed, a property file "dynamic.properties" appears and displays the date, time and count incrementing each time.
[java]
#Tue, 09 Feb 2016 12:48:20 -0700
date=2016/02/09 12\:48
buildnumber=3[/java]
Description
Version Control Systems allow to check in, check out, update the code from a build file. One such popular Version Control Systems is CVS. These are represented by a blue icon in the structure.
Examples
[java]
<?xml version=”1.0” encoding=”UTF-8”?>
<project name=”Version Control Examples” default=”cvsupdate” basedir=”.”>
<target name=”init” description=”Initialises properties”>
<property name=”src.dir” value=”src”/>
</target>
<target name=”cvsupdate” depends=”init”>
<cvs dest=”${src.dir}” comman=”update”/>
</target>
<target name=”checkout” depends=”init”>
<cvs cvsRoot=”:pserver:anoncvs@cvs.apache.org:/home/cvspublic” Package=”com/oncue/android/vocalease” Dest=”${src.dir}” />
</target>
<target name=”commandline”>
<cvs output=”patch”>
<commandline>
<argument value=”-q”/>
<argument value=”diff”/>
<argument value=”-u”/>
<argument value=”-N”/>
</commandline>
</cvs>
</target>
</project>
[/java]
Description
Junit tests can be run automatically using JUnit task, which is a part of the build process.
Examples
The structure of JUnit example is as follows:
junit.xml
[java]
<?xml version="1.0" encoding="UTF-8"?>
<project name="JUnit Example" default="test" basedir=".">
<target name="init">
<property name="src.dir" value="../src" />
<property name="reports.dir" value="reports" />
<property name="build.dir" value="build" />
<path id="junit.class.path">
<pathelement location="lib/junit.jar" />
<pathelement location="${build.dir}" />
</path>
</target>
<target name="compile" depends="init">
<javac srcdir="${src.dir}" destdir="${build.dir}" includeantruntime="false">
<classpath refid="junit.class.path" />
</javac>
</target>
<target name="test" depends="compile">
<junit printsummary="yes" haltonfailure="yes" showoutput="yes" >
<classpath refid="junit.class.path" />
<formatter type="xml" />
<batchtest fork="yes" todir="${reports.dir}/">
<formatter type="xml"/>
<fileset dir="${src.dir}">
<include name="*Test*.java"/>
</fileset>
</batchtest>
</junit>
</target>
<target name="report" depends="init">
<junitreport todir="${reports.dir}">
<fileset dir="${reports.dir}" includes="TEST-*.xml"/>
<report format="noframes" todir="${reports.dir}"/>
</junitreport>
</target>
</project>
[/java]
When this code is run,
TestMath.xml file is generated in reports directory.
[java]
<?xml version="1.0" encoding="UTF-8" ?>
<testsuite errors="0" failures="0" hostname="pentium4" name="TestMath" skipped="0" tests="2" time="0.405" timestamp="2014-06-05T23:49:34">
<properties>
<property name="java.vendor" value="Oracle Corporation" />
<property name="netbeans.user" value="C:\Documents and Settings\Rusty\Application Data\NetBeans\7.3.1" />
<property name="sun.java.launcher" value="SUN_STANDARD" />
<property name="sun.management.compiler" value="HotSpot Client Compiler" />
<property name="netbeans.autoupdate.version" value="1.23" />
<property name="os.name" value="Windows XP" />
<property name="sun.boot.class.path" value="C:\Program Files\Java\jdk1.7.0_25\jre\lib\resources.jar;C:\Program Files\Java\jdk1.7.0_25\jre\lib\rt.jar;C:\Program Files\Java\jdk1.7.0_25\jre\lib\sunrsasign.jar;C:\Program Files\Java\jdk1.7.0_25\jre\lib\jsse.jar;C:\Program Files\Java\jdk1.7.0_25\jre\lib\jce.jar;C:\Program Files\Java\jdk1.7.0_25\jre\lib\charsets.jar;C:\Program Files\Java\jdk1.7.0_25\jre\lib\jfr.jar;C:\Program Files\Java\jdk1.7.0_25\jre\classes" />
<property name="netbeans.importclass" value="org.netbeans.upgrade.AutoUpgrade" />
<property name="sun.desktop" value="windows" />
<property name="java.vm.specification.vendor" value="Oracle Corporation" />
<property name="ant.home" value="C:\apache-ant-1.9.4" />
<property name="java.runtime.version" value="1.7.0_25-b17" />
<property name="netbeans.default_userdir_root" value="C:\Documents and Settings\Rusty\Application Data\NetBeans" />
<property name="netbeans.accept_license_class" value="org.netbeans.license.AcceptLicense" />
<property name="user.name" value="Rusty" />
<property name="build.dir" value="build" />
<property name="nb.show.statistics.ui" value="usageStatisticsEnabled" />
<property name="user.language" value="en" />
<property name="sun.boot.library.path" value="C:\Program Files\Java\jdk1.7.0_25\jre\bin" />
<property name="reports.dir" value="reports" />
<property name="nb.native.filechooser" value="false" />
<property name="ant.project.default-target" value="test" />
<property name="ant.project.name" value="JUnit Example" />
<property name="java.version" value="1.7.0_25" />
<property name="sun.java2d.dpiaware" value="true" />
<property name="user.timezone" value="" />
<property name="sun.arch.data.model" value="32" />
<property name="java.endorsed.dirs" value="C:\Program Files\Java\jdk1.7.0_25\jre\lib\endorsed" />
<property name="sun.cpu.isalist" value="" />
<property name="netbeans.productversion" value="NetBeans IDE 7.3.1 (Build 201306052037)" />
<property name="sun.jnu.encoding" value="Cp1252" />
<property name="file.encoding.pkg" value="sun.io" />
<property name="file.separator" value="\" />
<property name="java.specification.name" value="Java Platform API Specification" />
<property name="java.class.version" value="51.0" />
<property name="user.country" value="US" />
<property name="java.home" value="C:\Program Files\Java\jdk1.7.0_25\jre" />
<property name="netbeans.buildnumber" value="201306052037" />
<property name="java.vm.info" value="mixed mode, sharing" />
<property name="org.openide.version" value="deprecated" />
<property name="ant.file" value="C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit\junit.xml" />
<property name="os.version" value="5.1" />
<property name="netbeans.system_http_proxy" value="DIRECT" />
<property name="netbeans.hash.code" value="unique=NB_CND_GFMOD_JAVA_MOB_PHP_WEBCOMMON_WEBEE0155732f5-0f2a-49cd-9089-5578abae1429_862843d1-e908-43d0-929e-4753d9b6c7c6" />
<property name="netbeans.autoupdate.country" value="US" />
<property name="sun.awt.keepWorkingSetOnMinimize" value="true" />
<property name="path.separator" value=";" />
<property name="java.vm.version" value="23.25-b01" />
<property name="user.variant" value="" />
<property name="netbeans.dynamic.classpath" value="C:\Program Files\NetBeans 7.3.1\platform\core\core.jar;C:\Program Files\NetBeans 7.3.1\platform\core\org-openide-filesystems.jar;C:\Program Files\NetBeans 7.3.1\platform\core\locale\core_ja.jar;C:\Program Files\NetBeans 7.3.1\platform\core\locale\core_pt_BR.jar;C:\Program Files\NetBeans 7.3.1\platform\core\locale\core_ru.jar;C:\Program Files\NetBeans 7.3.1\platform\core\locale\core_zh_CN.jar;C:\Program Files\NetBeans 7.3.1\platform\core\locale\org-openide-filesystems_ja.jar;C:\Program Files\NetBeans 7.3.1\platform\core\locale\org-openide-filesystems_pt_BR.jar;C:\Program Files\NetBeans 7.3.1\platform\core\locale\org-openide-filesystems_ru.jar;C:\Program Files\NetBeans 7.3.1\platform\core\locale\org-openide-filesystems_zh_CN.jar;C:\Program Files\NetBeans 7.3.1\nb\core\org-netbeans-upgrader.jar;C:\Program Files\NetBeans 7.3.1\nb\core\locale\core_nb.jar;C:\Program Files\NetBeans 7.3.1\nb\core\locale\org-netbeans-upgrader_ja.jar;C:\Program Files\NetBeans 7.3.1\nb\core\locale\org-netbeans-upgrader_pt_BR.jar;C:\Program Files\NetBeans 7.3.1\nb\core\locale\org-netbeans-upgrader_ru.jar;C:\Program Files\NetBeans 7.3.1\nb\core\locale\org-netbeans-upgrader_zh_CN.jar" />
<property name="jna.boot.library.name" value="jnidispatch-340" />
<property name="sun.awt.enableExtraMouseButtons" value="true" />
<property name="java.awt.printerjob" value="sun.awt.windows.WPrinterJob" />
<property name="ant.junit.enabletestlistenerevents" value="true" />
<property name="sun.io.unicode.encoding" value="UnicodeLittle" />
<property name="awt.toolkit" value="sun.awt.windows.WToolkit" />
<property name="netbeans.autoupdate.variant" value="" />
<property name="user.script" value="" />
<property name="org.openide.major.version" value="IDE/1" />
<property name="user.home" value="C:\Documents and Settings\Rusty" />
<property name="netbeans.home" value="C:\Program Files\NetBeans 7.3.1\platform" />
<property name="java.specification.vendor" value="Oracle Corporation" />
<property name="apple.laf.useScreenMenuBar" value="true" />
<property name="org.xml.sax.driver" value="com.sun.org.apache.xerces.internal.jaxp.SAXParserImpl$JAXPSAXParser" />
<property name="java.library.path" value="C:\Program Files\Java\jdk1.7.0_25\jre\bin;C:\WINDOWS\Sun\Java\bin;C:\WINDOWS\system32;C:\WINDOWS;C:\WINDOWS\system32;C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\Program Files\Common Files\iZotope\Runtimes;C:\Program Files\OpenLibraries\bin;C:\Program Files\Common Files\Roxio Shared\DLLShared\;C:\Program Files\Common Files\Roxio Shared\10.0\DLLShared\;C:\Program Files\QuickTime\QTSystem\;C:\Program Files\MySQL\MySQL Enterprise Backup 3.8.2\;%ANT_HOME\bin;C:\Program Files\CVSNT\;C:\Program Files\Java\jdk1.7.0_25\bin;C:\apache-ant-1.9.4\bin;C:\android-sdk-windows\tools;." />
<property name="java.vendor.url" value="http://java.oracle.com/" />
<property name="java.vm.vendor" value="Oracle Corporation" />
<property name="java.runtime.name" value="Java(TM) SE Runtime Environment" />
<property name="sun.java.command" value="org.apache.tools.ant.taskdefs.optional.junit.JUnitTestRunner TestMath skipNonTests=false filtertrace=true haltOnError=false haltOnFailure=true formatter=org.apache.tools.ant.taskdefs.optional.junit.SummaryJUnitResultFormatter showoutput=true outputtoformatters=true logfailedtests=true threadid=0 logtestlistenerevents=true formatter=org.apache.tools.ant.taskdefs.optional.junit.XMLJUnitResultFormatter,C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit\reports\TEST-TestMath.xml formatter=org.apache.tools.ant.taskdefs.optional.junit.XMLJUnitResultFormatter,C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit\reports\TEST-TestMath.xml crashfile=C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit\junitvmwatcher6703561272646677318.properties propsfile=C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit\junit9101159076003269994.properties" />
<property name="java.class.path" value="C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit\lib\junit.jar;C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit\build;C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit;C:\PROGRA~1\JMF21~1.1E\lib\sound.jar;C:\PROGRA~1\JMF21~1.1E\lib\jmf.jar;C:\PROGRA~1\JMF21~1.1E\lib;C:\;C:\junit3.8.1\junit.jar;C:\JNA\jna.jar;C:\apache-ant-1.9.4\lib\ant-launcher.jar;C:\apache-ant-1.9.4\lib\ant.jar;C:\apache-ant-1.9.4\lib\ant-junit.jar;C:\apache-ant-1.9.4\lib\ant-junit4.jar" />
<property name="ant.version" value="Apache Ant(TM) version 1.9.4 compiled on April 29 2014" />
<property name="sun.java2d.noddraw" value="true" />
<property name="jdk.home" value="C:\Program Files\Java\jdk1.7.0_25" />
<property name="java.vm.specification.name" value="Java Virtual Machine Specification" />
<property name="ant.file.JUnit Example" value="C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit\junit.xml" />
<property name="org.openide.specification.version" value="6.2" />
<property name="java.vm.specification.version" value="1.7" />
<property name="sun.cpu.endian" value="little" />
<property name="sun.os.patch.level" value="Service Pack 3" />
<property name="java.io.tmpdir" value="C:\DOCUME~1\Rusty\LOCALS~1\Temp\" />
<property name="java.vendor.url.bug" value="http://bugreport.sun.com/bugreport/" />
<property name="netbeans.dirs" value="C:\Program Files\NetBeans 7.3.1\nb;C:\Program Files\NetBeans 7.3.1\ergonomics;C:\Program Files\NetBeans 7.3.1\ide;C:\Program Files\NetBeans 7.3.1\java;C:\Program Files\NetBeans 7.3.1\apisupport;C:\Program Files\NetBeans 7.3.1\webcommon;C:\Program Files\NetBeans 7.3.1\websvccommon;C:\Program Files\NetBeans 7.3.1\enterprise;C:\Program Files\NetBeans 7.3.1\mobility;C:\Program Files\NetBeans 7.3.1\profiler;C:\Program Files\NetBeans 7.3.1\python;C:\Program Files\NetBeans 7.3.1\php;C:\Program Files\NetBeans 7.3.1\identity;C:\Program Files\NetBeans 7.3.1\harness;C:\Program Files\NetBeans 7.3.1\cnd;C:\Program Files\NetBeans 7.3.1\dlight;C:\Program Files\NetBeans 7.3.1\groovy;C:\Program Files\NetBeans 7.3.1\extra;C:\Program Files\NetBeans 7.3.1\javacard;C:\Program Files\NetBeans 7.3.1\javafx" />
<property name="netbeans.autoupdate.language" value="en" />
<property name="java.awt.graphicsenv" value="sun.awt.Win32GraphicsEnvironment" />
<property name="os.arch" value="x86" />
<property name="java.util.logging.config.class" value="org.netbeans.core.startup.TopLogging" />
<property name="java.ext.dirs" value="C:\Program Files\Java\jdk1.7.0_25\jre\lib\ext;C:\WINDOWS\Sun\Java\lib\ext" />
<property name="user.dir" value="C:\Program Files\NetBeans 7.3.1" />
<property name="build.compiler.emacs" value="true" />
<property name="line.separator" value="
" />
<property name="apple.awt.graphics.UseQuartz" value="true" />
<property name="java.vm.name" value="Java HotSpot(TM) Client VM" />
<property name="basedir" value="C:\Documents and Settings\Rusty\My Documents\NetBeansProjects\Ant\junit" />
<property name="sun.awt.datatransfer.timeout" value="1000" />
<property name="ant.java.version" value="1.7" />
<property name="ant.file.type.JUnit Example" value="file" />
<property name="ant.core.lib" value="C:\apache-ant-1.9.4\lib\ant.jar" />
<property name="file.encoding" value="Cp1252" />
<property name="java.specification.version" value="1.7" />
<property name="src.dir" value="../src" />
<property name="ant.project.invoked-targets" value="test" />
</properties>
<testcase classname="TestMath" name="testMulitply" time="0.0" />
<testcase classname="TestMath" name="testAdd" time="0.0" />
<system-out><![CDATA[]]></system-out>
<system-err><![CDATA[]]></system-err>
</testsuite>
[/java]
This is not in human readable format. Hence, the junit.xml depicts the reports file using fileset. This concept will be explained in the coming lessons.
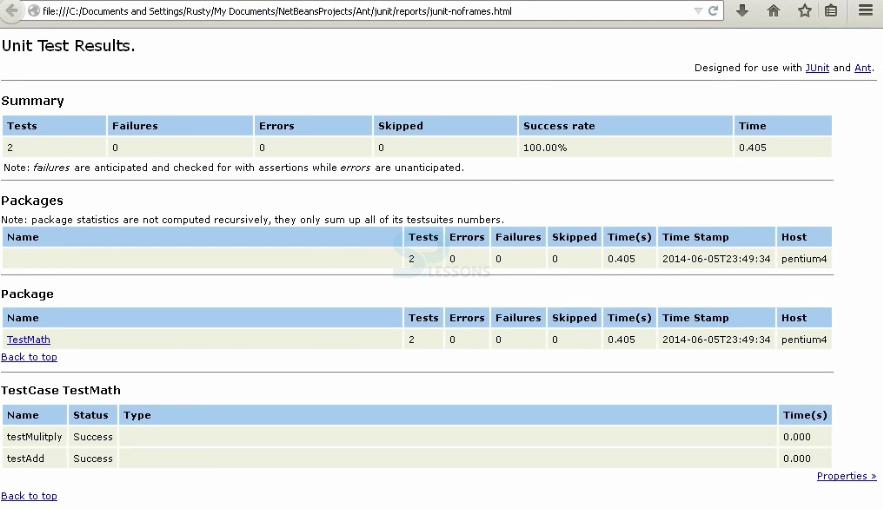
Description
It is required to deploy the files to other machines. If it is a local machine, copy task is enough but if it is a remote machine, FTP task must be used.
Examples
deploy.xml
[java]
<?xml version="1.0" encoding="UTF-8"?>
<project name="Deploy Example" default="ftp" basedir=".">
<target name="init">
<property name="server.name" value="your.server.com" />
<property name="remote.dir" value="incoming" />
<property name="user.id" value="your.email" />
<property name="password" value="your.password" />
<property name="deploy.dir" value="deploy" />
<property name="docs.dir" value="docs" />
</target>
<target name="copy" depends="init">
<copy file="${docs.dir}/test.html" todir="${deploy.dir}" />
</target>
<target name="ftp" depends="init">
<ftp server="${server.name}" remotedir="${remote.dir}" userid="${user.id}" password="${password}" passive="yes" binary="no" verbose="yes">
<fileset dir="${docs.dir}">
<include name="*.html"/>
</fileset>
</ftp>
</target>
<target name="get" depends="init">
<ftp action="get" server="ftp.apache.org" userid="anonymous" password="me@myorg.com">
<fileset dir="${docs}">
<include name="*.html"/>
</fileset>
</ftp>
</target>
[/java]
Appropriate jar files must be included and the code has to be run on ftp server which then gives the following output by transferring the file.

Key Points
- Archive Task helps to wrap the code using jar, war, and ear files.
- File tasks include copy, move, concat, sync, get, move, and replace.
- Property elements makes the code reusable.
- Version Control Systems are represented by a blue icon.
- Remote tasks uses FTP Server.